This homework is useing dartpad, so if u don’t use dartpad, please don’t pick up this work.
ANSWER
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Flutter Widget Showcase'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Welcome to my Flutter app!'),
SizedBox(height: 20),
Icon(Icons.star, size: 50, color: Colors.orange),
SizedBox(height: 20),
RaisedButton(
onPressed: () {
// Button onPressed action
},
child: Text('Raised Button'),
),
SizedBox(height: 20),
FlatButton(
onPressed: () {
// Button onPressed action
},
child: Text('Flat Button'),
),
SizedBox(height: 20),
Image.network(
'https://via.placeholder.com/150',
width: 150,
height: 150,
),
SizedBox(height: 20),
TextField(
decoration: InputDecoration(
hintText: 'Enter text here',
labelText: 'Text Field',
),
),
SizedBox(height: 20),
Container(
width: 100,
height: 100,
color: Colors.blue,
),
SizedBox(height: 20),
Checkbox(
value: false,
onChanged: (bool newValue) {
// Checkbox onChanged action
},
),
SizedBox(height: 20),
Radio(
value: 1,
groupValue: 1,
onChanged: (int? value) {
// Radio onChanged action
},
),
SizedBox(height: 20),
Switch(
value: false,
onChanged: (bool newValue) {
// Switch onChanged action
},
),
],
),
),
);
}
}
This Flutter app consists of an AppBar at the top and a variety of widgets in the body, including text, icons, buttons, an image, a text field, a container, a checkbox, a radio button, and a switch. You can customize the widgets and their functionality as needed.
QUESTION
Description
Create any Flutter app with at least 10 different widgets. Your app has to have a scaffold and an app bar.
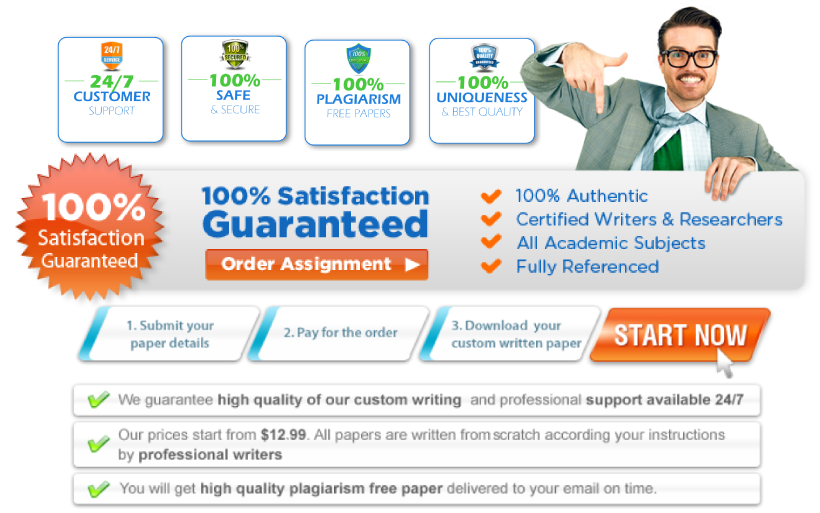