SFSU Write a Currency Conversion GUI Application Programming Task
ANSWER
Creating a currency conversion GUI application in Java Swing requires several steps. Here’s a basic outline of how you can build such an application. You will need Java and the Swing library installed on your system.
- Set Up Your Project: Create a new Java project in your favorite Integrated Development Environment (IDE) and add the necessary Swing libraries to your project.
- Design the GUI: Create the GUI components, including text fields, labels, and buttons. You will also need to create labels for currency icons using JLabels. You can use ImageIcon to load the currency icons.
java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;public class CurrencyConverterApp extends JFrame {
private JTextField inputTextField;
private JTextField resultTextField;
private JButton convertButton;
private JLabel fromCurrencyLabel;
private JLabel toCurrencyLabel;
private JLabel fromCurrencyIconLabel;
private JLabel toCurrencyIconLabel;public CurrencyConverterApp() {
// Set up the main frame
setTitle("Currency Converter");
setSize(400, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new GridLayout(3, 3));// Create and add components to the frame
inputTextField = new JTextField();
resultTextField = new JTextField();
resultTextField.setEditable(false);
convertButton = new JButton("Convert");
fromCurrencyLabel = new JLabel("From Currency:");
toCurrencyLabel = new JLabel("To Currency:");
fromCurrencyIconLabel = new JLabel(new ImageIcon("from_currency_icon.png"));
toCurrencyIconLabel = new JLabel(new ImageIcon("to_currency_icon.png"));// Add components to the frame
add(fromCurrencyLabel);
add(inputTextField);
add(fromCurrencyIconLabel);
add(toCurrencyLabel);
add(resultTextField);
add(toCurrencyIconLabel);
add(convertButton);// Attach an action listener to the Convert button
convertButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
// Perform the currency conversion here
double inputValue = Double.parseDouble(inputTextField.getText());
// Call a currency conversion method here and update resultTextField
double convertedValue = convertCurrency(inputValue);
resultTextField.setText(String.valueOf(convertedValue));
}
});
}// Implement the currency conversion method
private double convertCurrency(double inputValue) {
// Connect to the currency conversion webpage and parse the result
// Return the converted value
return inputValue * exchangeRate; // Replace with actual exchange rate
}public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
new CurrencyConverterApp().setVisible(true);
}
});
}
}
- Currency Conversion Logic: Implement the
convertCurrency
method, which will connect to the currency conversion webpage you mentioned, fetch the exchange rate for the selected currencies, and perform the conversion. - Run the Application: In the
main
method, create an instance of yourCurrencyConverterApp
class and make it visible. - Styling and Layout: You can further style and format the GUI components to match your desired appearance.
- Currency Icons: Replace
"from_currency_icon.png"
and"to_currency_icon.png"
with the actual paths to your currency icon images. - Currency Conversion API: You’ll need to integrate a currency conversion API to get real-time exchange rates. You can use various APIs available online, such as Open Exchange Rates or CurrencyLayer. Replace
exchangeRate
in theconvertCurrency
method with the actual exchange rate obtained from the API. - Error Handling: Add error handling for cases where the user enters invalid input or if the currency conversion fails.
This is a basic template for creating a currency conversion GUI application in Java Swing. You can enhance it by adding features like currency selection, historical exchange rate data, and error handling for network connectivity issues.
QUESTION
Description
Write a currency-conversion GUI application as shown in the picture. The input currency should be entered from the keyboard (via a JTextField). A read-only JTextField should be used to display the converted currency. Use given images to create curreny icons using JLabels. The application should allow the user to make coversion between any two types.
Use the following webpage for the currency conversion:
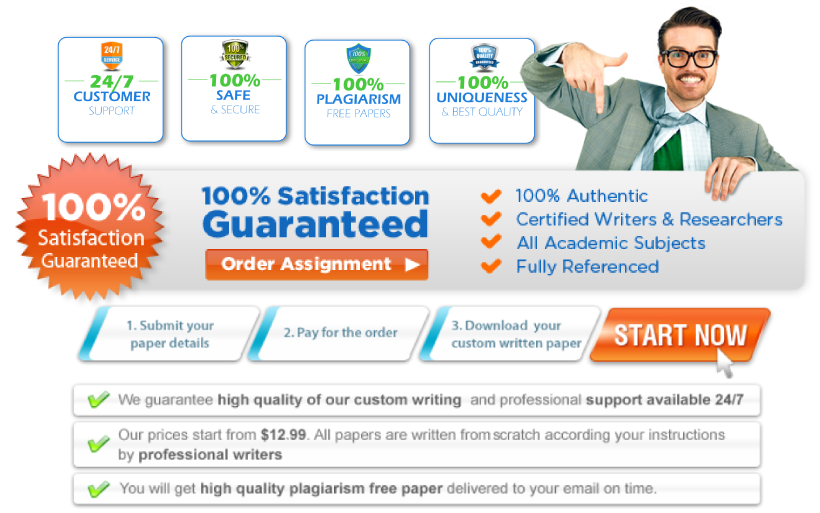