NVCC Programming Dynamic Memory Management Schemes Project
ANSWER
Creating a complete implementation of the malloc
, free
, calloc
, and realloc
functions, along with the requested features, is a complex task that involves a significant amount of code. I can provide you with a high-level outline of how you can approach this task and some important concepts you’ll need to understand. You will need to write the actual code based on this outline.
Here is a simplified outline:
- Implementing a Memory Manager:
- You will need to maintain a data structure to manage the allocated and free blocks of memory. This data structure will be used to keep track of available memory and allocate memory when requested.
- Implement
malloc
Functionality:- Allocate memory from the available free blocks. You will need to search for a suitable free block based on the requested size.
- If the free block is larger than the requested size, split it into two blocks: one for the requested size and another for the remaining free memory.
- Implement
free
Functionality:- When
free
is called, mark the allocated block as free. You should also merge adjacent free blocks to avoid fragmentation.
- When
- Implement
calloc
Functionality:- Implementing
calloc
is similar tomalloc
, but you also need to initialize the allocated memory to zero.
- Implementing
- Implement
realloc
Functionality:realloc
involves changing the size of an already allocated block. You may need to allocate a new block, copy data from the old block to the new one, and then free the old block.
- Tracking Memory Leaks:
- To track memory leaks, you should keep a record of all allocated blocks. When the program exits, you can check this record to see if any blocks were not freed.
- Implementing a Doubly Linked List:
- Modify the memory manager data structure to use a doubly linked list to keep track of free and allocated blocks.
- Implementing Best Fit Algorithm:
- Modify the allocation strategy to use the best fit algorithm. This involves finding the smallest free block that can accommodate the requested size.
- Bonus Points:
- To minimize wasted space, you can implement a function to split blocks into smaller ones when they are larger than needed.
- Implement a mechanism to merge adjacent free blocks together to avoid fragmentation.
- Testing and Profiling:
- Write a
main
function to test your memory manager. Allocate and free memory blocks multiple times to test various scenarios. - Measure memory usage and fragmentation to demonstrate the efficiency of your implementation.
- Write a
- Reporting:
- Create a report that explains your implementation, highlights any issues or limitations, and presents memory leak statistics for different test cases.
Remember that this is a complex task, and you will need a good understanding of memory management and data structures to complete it successfully. You can refer to the tutorial you mentioned for guidance on implementing the basic memory manager functions and adapt them to meet the requirements of your assignment.
QUESTION
Description
Develop a C program to implement the “malloc”, “free”, “calloc” and “realloc” functionalities.
INSTRUCTIONS:
1. Check out the malloc tutorial from Dan Luu on how these functions can be implemented at:
http://danluu.com/malloc-tutorial/
The tutorial implements working versions of malloc, free, calloc and realloc and you can use that code as-is.
2. Write a driver function (i.e., main function) to demonstrate the usage and efficiency of these functions.
The main() should have at least 10 calls each to malloc, calloc, realloc and free. Set up the function to (i) print out
the heap start/end addresses and (ii) print out the memory leaks in your code. In your report, explain how you
calculated memory leaks in this case.
3. Your main task is to implement the exercises mentioned in the tutorial. These are also shown below:
(a) Convert the single linked list implementation of the tutorial into a doubly linked list version; make changes to
all the functions accordingly to work with the doubly linked list.
(b) The current implementation implements a first fit algorithm for finding free blocks. Implement a best fit
algorithm instead.
4. Your code must use the set-up mentioned in this tutorial. Other online resources can be consulted but
NOT copied. The tutorial mentions some other implementations for splitting/merging blocks that can only be
consulted but not copied.
To turn in:
1. You will need 4 files: (a) a header file with all the function prototypes, (b) a .c file with all the function
definitions, (c) a .c file with the driver main() function, (d) a Makefile to compile your code and (e) a report
showing the memory leak values. Submit both the improved versions of the functions and the preliminary
implementations from the tutorial. Additionally, mention which portions of your code are working and which
portions don’t and why in your report. Your report should show the memory leaks for varying number of calls to
malloc/calloc/realloc/free.
Bonus Points
(a) The implemented malloc is really wasteful if we try to re-use an existing block and we don’t need all of the
space. Implement a function that will split up blocks so that they use the minimum amount of space necessary.
(b) After doing (a), if we call malloc and free lots of times with random sizes, we’ll end up with a bunch of small
blocks that can only be re-used when we ask for small amounts of space. Implement a mechanism to merge
adjacent free blocks together so that any consecutive free blocks will get merged into a single block.
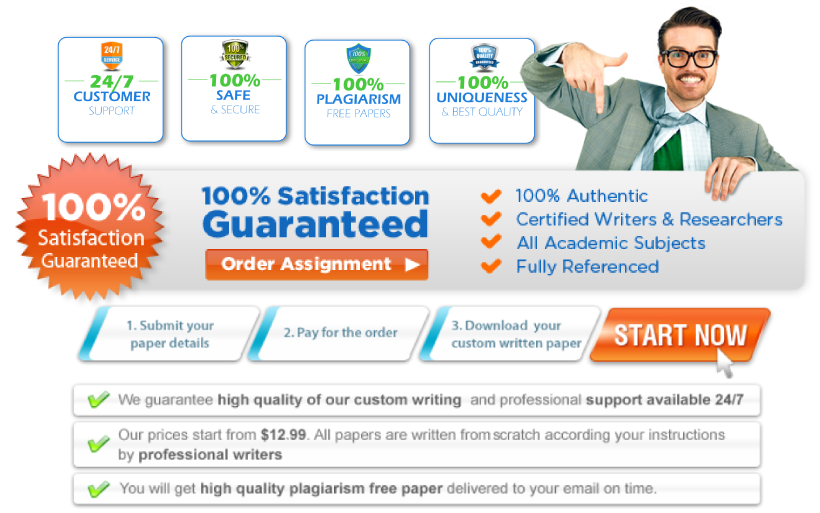