Data Abstract & Structures Trees Worksheet
ANSWER
8.3 Lab: BT – In-, Post-, and Pre-Order Traversals:
Implementing in-order, post-order, and pre-order traversal functions for a binary tree is the task in this assignment. For every traversal type, you must construct private recursive and public wrapper functions that invoke these private functions. Here’s a broad synopsis:
Provide the required data members and methods in your Binary Tree class definition.
Implement the private recursive functions _inOrder, _postOrder, and _preOrder for every kind of traversal. These functions should carry out the traverse with the current node as an argument.
Implement the pre-order, post-order, and in-order public wrapper methods. Starting with the root node, these functions should call their corresponding private counterparts.
8.4 Lab: Version 2 of BT – Traversals (in-, post-, and pre-order)
The usage of function pointers for traversals is introduced in this version. You’ll need to alter the traversal functions to accept a function pointer that indicates how to handle the data during traversal. This is what you ought to do:
To accept a function pointer as a parameter, change the method signatures for inOrder, _inOrder, postOrder, _postOrder, preOrder, and _preOrder.
Use the supplied function pointer to apply an action to the data at each node during traversal rather than processing the data directly.
Pass in the function pointer that describes the intended behavior during traversal when invoking these functions from the public wrapper functions.
8.13 Lab: BST (Traversals) <— BT:
Rewriting a Binary Tree class to serve as a parent class for a Binary Search Tree class is the task at hand. You are required to implement traversals for the BST that are both pre-order and post-order. They are as follows:
Construct a class called Binary Search Tree, which is derived from the Binary Tree class.
Use the same methodology as in 8.3 Lab to implement the Binary Tree class’s post-order and pre-order traversal functions.
If required, override the Binary Search Tree class’s traversal functions.
Lab 8.14: BT <— BST (Largest/Smallest):
It would help if you recursively implemented functions to determine the lowest and largest elements in a BST for this assignment. Observe these steps:
Create the findSmallest function, which recursively looks for the tree’s smallest element.
Create the findLargest function, which recursively looks for the largest entry in the tree.
The root node should be the starting point for these functions’ searches.
8.15 Lab: BST (Indented Tree) <— BT
It would help if you created a depth-first traversal variant that shows an indented tree with level numbers to complete this job. This is the way to go about it:
Create a depth-first traversal function, such as printTree, that accepts a function to handle the output, a level number, and the current node.
Use recursion to visit each node in this traversal and then display the value of each node with the proper indentation according to the level number.
From your main program, call the printTree function with the root node and an initial level number.
8.16 Lab: BST (Search) <— BT:
It would help if you implemented search functions for the BST in this assignment. It can be done as follows:
Create a private recursive _search function that accepts a current node and a value to search for. If the value is found, this method should iterate through the tree and return true; if not, it should return false.
Create a public search function that serves as _search’s wrapper. The search should begin at the root node and return the result.
QUESTION
Description
8.3 Lab: BT – Traversals (in-, post-, and pre-Order)
This program builds a Binary Tree of random integers and displays the tree in inorder.
Note The insert()
function is specific to this exercise
The main goal of this example is build a binary tree that could be used to test the binary tree traversals and other binary tree functions.
Implement the postorder and preorder traversals.
Note: For each traversal there are two functions:
- a recursive private function that traverses the binary tree: because it needs the root as a parameter, it cannot be called outside of the class, therefore it has to be declared private
- a public function (the wrapper) that calls the private traversal
Naming conventions: usually private member functions’ names begin with ‘_’
inOrder() calls _inOrder()
postOrder() calls _postOrder()
preOrder() calls _preOrder(
8.4 Lab: BT – Traversals (in-, post-, and pre-Order) – Version 2
This program builds a Binary Tree of random integers and displays the tree in inorder.
Our final goal is to write binary tree and binary search tree ADTs. We’ve seen one way to deal with traversal in the Doubly-Linked-List ADT assignment: overload the stream insertion operator. This gives us only one way of formatting the output. The approach that we will use with trees is a more flexible approach that gives us more formatting options, but requires the use of function pointers.
This assignment gives you an example of how function pointers can be used to write ADTs. You will learn more about this topic in an Advanced C/C++ class.
The inorder traversal functions have been changed from:
void inOrder() const;
void _inOrder(Node *root) const;
to:
void inOrder( void visit(const Data &) ) const;
void _inOrder(Node *root, void visit(const Data &) ) const;
The parameter that has been added to both function:
void visit(const Data &)
looks like a function’s prototype declaration, but it represents a function parameter (or a function pointer). The name of a function represents an address (pointer constant), and this is a value that we can pass to other functions.
Review the inorder traversal and implement the postorder and preorder traversals using function pointers.
8.13 Lab: BT <— BST (Traversals)
Review Inheritance (A search tree is a binary tree)
In this assignment we will rewrite the Binary Tree class as a parent class for the Binary Search Tree class. The tree node is also a class.
The assignment consists of the following classes/files:
- BinaryNode.h (template, given)
- BinaryTree.h (template, incomplete)
- BinarySearchTree.h(template, incomplete)
- main.cpp (incomplete)
The program creates a BST of random integers. The insert and inorder traversal are given.
Write the following functions:
- postorder traversal
- preorder traversal
8.14 Lab: BT <— BST (Smallest/Largest)
The assignment consists of the following classes/files:
- BinaryNode.h (template, given)
- BinaryTree.h (template, incomplete)
- BinarySearchTree.h(template, incomplete)
- main.cpp (incomplete)
Write the following functions:
- findSmallest (recursive)
- findLargest (recursive)
8.15 Lab: BT <— BST (Indented Tree)
Review Inheritance (A search tree is a binary tree)
In this assignment we will rewrite the Binary Tree class as a parent class for the Binary Search Tree class. The tree node is also a class.
The assignment consists of the following classes/files:
- BinaryNode.h (template, given)
- BinaryTree.h (template, incomplete)
- BinarySearchTree.h(template, incomplete)
- main.cpp (incomplete)
The program creates a BST of random integers. The insert and inorder traversal are given.
Write a variation of one of the Depth-First Traversal Functions named printTree that displays the indented tree, including the level numbers.
If the three has 3 nodes: 27, 25, and 30:
27
/ \
25 30
the indented tree will be displayed as shown below:
1). 27
..2). 30
..2). 25
8.16 Lab: BT <— BST (Search)
In this assignment we will rewrite the Binary Tree class as a parent class for the Binary Search Tree class. The tree node is also a class.
The assignment consists of the following classes/files:
- BinaryNode.h (template, given)
- BinaryTree.h (template, incomplete)
- BinarySearchTree.h(template, incomplete)
- main.cpp (incomplete)
The program creates a BST of random integers. The insert and inorder traversal are given.
Implement the pair of search functions for searching the BST:
_search
– recursive (private function)search
– wrapper for_search
(public function)
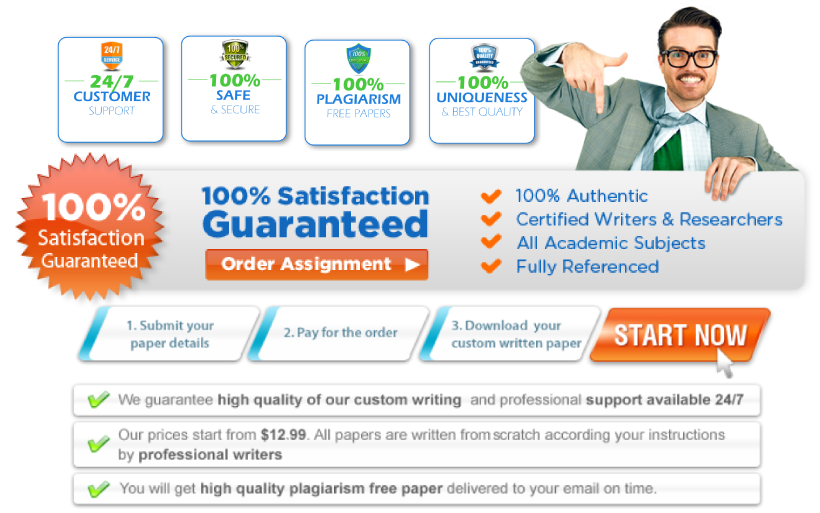