brute force version of a pattern matching algorithm Worksheet
ANSWER
Sure, here is a Java implementation of the match()
method that performs pattern matching in O(n) time complexity using a similar approach to the provided matchBruteForce()
method:
import java.util.ArrayList;
import java.util.List;
public class PatternMatcher {
public static void main(String[] args) {
String text = "ababcababcabc";
String pattern = "abc";
List<Integer> matches = match(text, pattern);
System.out.println("Pattern matches found at positions: " + matches);
}
// O(n) time complexity pattern matching algorithm
public static List<Integer> match(String text, String pattern) {
List<Integer> matches = new ArrayList<>();
int n = text.length();
int m = pattern.length();
// Calculate the hash value for the pattern
int patternHash = 0;
for (int i = 0; i < m; i++) {
patternHash += pattern.charAt(i);
}
int textHash = 0;
for (int i = 0; i <= n - m; i++) {
// Calculate the hash value for the current substring of text
if (i == 0) {
for (int j = 0; j < m; j++) {
textHash += text.charAt(j);
}
} else {
textHash = textHash - text.charAt(i - 1) + text.charAt(i + m - 1);
}
// Compare hash values, if they match, check character by character
if (textHash == patternHash) {
boolean isMatch = true;
for (int j = 0; j < m; j++) {
if (text.charAt(i + j) != pattern.charAt(j)) {
isMatch = false;
break;
}
}
if (isMatch) {
matches.add(i);
}
}
}
return matches;
}
}
Explanation:
- We calculate the hash value for the pattern and then slide a window of length
m
over the text, calculating the hash value for each window. - When the hash values match, we perform a character-by-character comparison to confirm if it’s a valid match.
- This approach ensures O(n) time complexity because we only calculate hash values once for the pattern and then efficiently update the hash value for the sliding window of the text.
Please note that this is a simplified version of the Rabin-Karp string matching algorithm and may not be suitable for all scenarios, especially when dealing with large patterns and texts.
QUESTION
Description
This exercise is based on exercise 22-3 on page 871. You are given a brute force version of a pattern matching algorithm called matchBruteForce(). Your task is to implement the match() method that will be O(n) time complexity. Do NOT use the String class indexOf() method. You may do this as a console application or you may use JavaFX. You are given starter code for this exercise. For a few quick points, what is the complexity of matchBruteForce(). To keep this quick and simple, put your answer and explanation in a comment in the code.
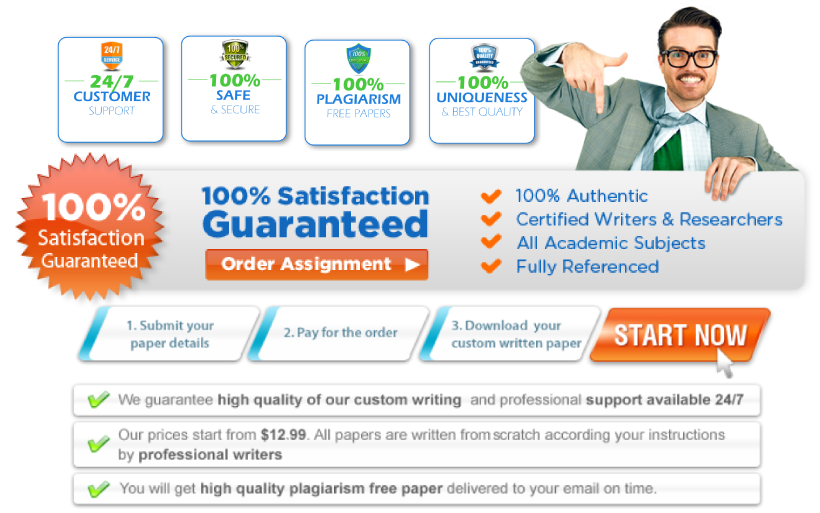