AU Programming Javascript Objects Project
ANSWER
- Create an HTML file (e.g.,
login.html
):
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Login Page</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="container">
<h2>Login</h2>
<form id="login-form">
<div class="form-group">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
</div>
<div class="form-group">
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
</div>
<button type="submit">Login</button>
</form>
<div id="login-status" class="hidden">
<p id="login-message"></p>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
- Create a CSS file (e.g.,
style.css
) to style the login form:
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.container {
background-color: #fff;
padding: 20px;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.2);
text-align: center;
}
.form-group {
margin-bottom: 10px;
}
label {
display: block;
font-weight: bold;
}
input[type="text"],
input[type="password"] {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 3px;
}
button {
background-color: #007bff;
color: #fff;
border: none;
padding: 10px 20px;
border-radius: 3px;
cursor: pointer;
}
.hidden {
display: none;
}
- Create a JavaScript file (e.g.,
script.js
) to handle the login logic:
document.addEventListener('DOMContentLoaded', function () {
const loginForm = document.getElementById('login-form');
const loginStatus = document.getElementById('login-status');
const loginMessage = document.getElementById('login-message');
const users = [
{ username: 'user1', password: 'password1' },
{ username: 'user2', password: 'password2' },
// Add more users as needed
];
loginForm.addEventListener('submit', function (e) {
e.preventDefault();
const usernameInput = document.getElementById('username').value;
const passwordInput = document.getElementById('password').value;
const user = users.find(u => u.username === usernameInput);
if (user && user.password === passwordInput) {
// Successful login
loginMessage.textContent = 'Login successful!';
loginMessage.style.color = 'green';
} else {
// Incorrect username or password
loginMessage.textContent = 'Incorrect username or password.';
loginMessage.style.color = 'red';
}
loginStatus.classList.remove('hidden');
});
});
In this example, we have a simple HTML form for the login page, a CSS file for styling, and a JavaScript file for handling the login logic. You can add more users to the users
array in the JavaScript file as needed. When a user submits the form, the script checks the username and password against the stored objects and displays a success or error message accordingly.
QUESTION
Description
I need a simple log in window where user can log in, we do not have to connect to any database, you can add the login details in the same files using javascript objects,on success log in, display another window showing success, when password and username do not mutch tell the user incorrect password
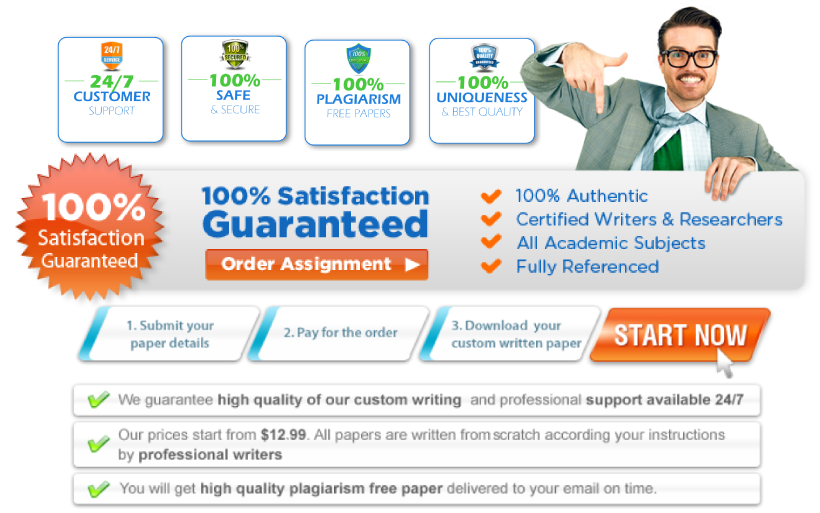